Step 1 - Create a frame
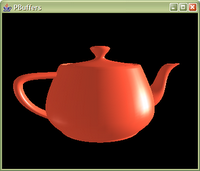
There are two types of OpenGL "windows" in JOGL, a GLCanvas and a GLJPanel. The GLJPanel is for use with the normal Java2D API while the GLCanvas is a high performace version. To create an OpenGL window first create a Frame and the simply add a GLCanvas to it.
Frame frame = new Frame("PBuffers") ;
GLCanvas canvas = new GLCanvas() ;
canvas.addGLEventListener(this);
frame.add(canvas);
frame.setSize(640, 480);
The application is notified of OpenGL events via 4 simple callbacks that are called following the registration of the GLEventListener listener via the canvas.addGLEventListener(this) call. It's easier to use the GLU functions with can be called from the GLU object.
Step 2 - Initilize the OpenGL context
The init() method is called when the windows is first created. The display() function is only called when the display needs to be repainted so the Animator class be used to update the display periodically.
public void init(GLAutoDrawable drawable)
{
final GL gl = drawable.getGL();
gl.setSwapInterval(0);
animator = new Animator(canvas);
animator.setRunAsFastAsPossible(true) ;
animator.start() ;
}
Step 3 - Add a display callback
The display() method is called when the window needs to be updated and is where all the OpenGL drawing should take place.
public void display(GLAutoDrawable drawable)
{
GL gl = drawable.getGL() ;
}
Step 4 - Add a reshape callback
The reshape() method is called when the window size changes. This callback is called once when the application is started.
public void reshape(GLAutoDrawable drawable, int x, int y, int width, int height)
{
GL gl = drawable.getGL();
float h = (float) width / (float) height;
gl.glMatrixMode(GL.GL_PROJECTION);
gl.glLoadIdentity();
glu.gluPerspective( 40.0, h, 1.00, 100.0 );
}
Step 5 - Add a displayChanged callback
The displayChanged() method is called then the display is changed.
public void displayChanged(GLAutoDrawable drawable, boolean modeChanged, boolean deviceChanged)
{
}
Notes:
- If the GLUT objects appear to be rendered back to front (or inside out) then try gl.glEnable(GL.GL_CW) as GLUT polygons are rendered in clock-wise order.
- Setup light sources with all options as LIGHT0 has defaults that the others lights do not appear to have.
gl.glLightfv(GL.GL_LIGHT1, GL.GL_POSITION, light2pos, 0);
gl.glLightfv(GL.GL_LIGHT1, GL.GL_AMBIENT, light2amb, 0);
gl.glLightfv(GL.GL_LIGHT1, GL.GL_DIFFUSE, light2diff, 0);
gl.glLightfv(GL.GL_LIGHT1, GL.GL_SPECULAR, light2spec, 0);
gl.glEnable(GL.GL_LIGHT1);